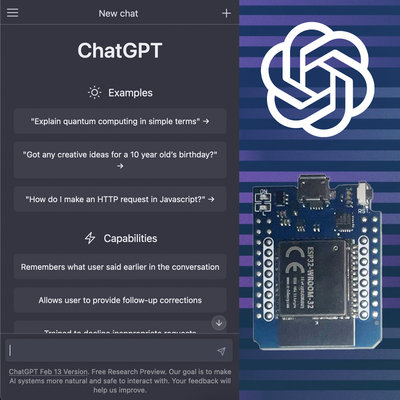
Use ChatGPT in ESP32 Microcontroller with OpenAI API
Add natural language processing capabilities to your ESP32 project by integrating the ChatGPT using OpenAI API. Step-by-step instructions with code examples
Introduction: Using ChatGPT to Enhance ESP32 Microcontrollers for IoT Projects #
ESP32 series of microcontrollers have become popular among IoT enthusiasts and hobbyists due to their low power consumption, built-in WiFi and Bluetooth connectivity, and powerful processing capabilities. With the addition of ChatGPT, you can create more sophisticated and interactive applications, such as chatbots or voice assistants, that can generate human-like responses to text input.
In this article, we'll explore the potential of using ChatGPT on ESP32 microcontrollers and go through instructions on how to set it up. We'll also review the benefits and challenges of this integration.
What is ChatGPT? #
ChatGPT is an advanced natural language processing (NLP) model developed by OpenAI that can generate human-like responses to your provided text. It uses a machine learning algorithm, which allows it to understand the context and meaning of text and remember what was said before.
You probably heard about it by now, as ChatGPT has gained 100 million users in 2 months since launch, making it the fastest-growing consumer app ever and has been trending all over the internet recently.
While ChatGPT was originally designed for conversational applications such as chatbots, but integrating ChatGPT with IoT devices, can bring more sophisticated devices, that can understand and respond to natural language input.
For example, imagine you have a smart home system that can control various devices such as lights, thermostats, and security cameras. Instead of using a mobile app or voice commands, you could use ChatGPT to communicate with the system using natural language with context. You could tell the device "I am feeling a bit hot" and it would check the temperature in your room if it is too high, it would suggest increasing it and if you have your windows open, could also suggest closing them.
What you need to know about ChatGPT API before you start developing #
If you haven't used ChatGPT API before, or just played around a little bit and did not check the documentation, there are a few things to keep in mind when using the ChatGPT API.
- 🔠 Character Limit: The maximum length of response text that can be generated by the API depends on the pricing plan that you have subscribed to. For example, the free plan has a limit of 2048 characters per request, while the paid plans can handle larger amounts of text. Be sure to check the documentation and plan details before using the API.
- 💲 Pricing: OpenAI's pricing for the ChatGPT API can be quite expensive, especially for larger volumes of text or higher frequency of requests. Be sure to review the pricing plans and any potential costs before integrating the API into your project.
- ⚖️ Ethics and Bias: It is important to be aware of the ethical implications of using such technology as ChatGPT. AI-generated text can have inherent biases based on the training data it was trained on, which can lead to discriminatory language or harmful stereotypes. It is important to use the API responsibly and with a critical eye towards the generated text.
- 🔌 API Availability: OpenAI's ChatGPT API is generally reliable, but there may be occasional some downtime or maintenance periods that can affect the availability of the API. It is important to have a backup plan in case the API becomes temporarily unavailable.
It's worth noting that OpenAI provides a free tier for its GPT-3 API, which allows for up to 100,000 tokens per month. However, if you require more than that, you may need to upgrade to a paid plan. A token can represent a word or a punctuation mark or symbol, such as a comma or period. Therefore, the number of words represented by 100,000 tokens can vary depending on the language and text being analyzed.
You can find more information on the OpenAI's website:
- 🔗 OpenAI API pricing page: https://openai.com/pricing
- 🔗 OpenAI API documentation: https://platform.openai.com/docs/api-reference/introduction
Using ChatGPT with ESP32 #
Integrating ChatGPT with ESP32 microcontrollers can open a place for a wide range of improved IoT applications, such as chatbots, voice assistants, and natural language interfaces. We will use the ChatGPT API in ESP32.
Here are the steps that we will need to do to get responses from ChatGPT in your application using ESP32 microcontroller.
- Sign up on an OpenAI website and install the necessary libraries on the ESP32.
- Create a new project on the OpenAI API and generate an API key.
- Use the API key to authenticate requests to the OpenAI API.
- Send text input to the OpenAI API using HTTP requests and receive a response in JSON format.
- Parse the response and use it to control the ESP32 microcontroller.
Code example to call ChatGPT API from ESP32 #
At this stage, you should have the ESP32 Arduino Core library installed and have the OpenAI API key ready.
- First, we need to include the necessary libraries for the ESP32 to communicate over Wi-Fi, make HTTP requests, and parse JSON data.
#include <WiFi.h>
#include <HTTPClient.h>
#include <ArduinoJson.h>
- Define the network credentials for the Wi-Fi network that the ESP32 will connect to, and the API key for the OpenAI API.
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
const char* apiKey = "your_API_KEY";
Note that the ssid
, password
, and apiKey
values should be replaced with the appropriate values of your network and OpenAI API key.
- Define the
setup()
function is where the ESP32 connects to the Wi-Fi network and sends an HTTP POST request to the OpenAI API.
void setup() {
//
}
- Inside the
setup()
function, we will first initialize Serial port.
// Initialize Serial
Serial.begin(9600);
- Next, we will connect to the WiFi network
// Connect to Wi-Fi network
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
- Continue filling in the
setup()
function to send the HTTP Post request to the OpenAI API endpoint for the completions endpoint, using theHTTPClient
library.
// Send request to OpenAI API
String inputText = "Hello, ChatGPT!";
String apiUrl = "https://api.openai.com/v1/completions";
String payload = "{\"prompt\":\"" + inputText + "\",\"max_tokens\":100, \"model\": \"text-davinci-003\"}";
HTTPClient http;
http.begin(apiUrl);
http.addHeader("Content-Type", "application/json");
http.addHeader("Authorization", "Bearer " + String(apiKey));
The inputText
string defines the prompt for the API, which in this example is "Hello, ChatGPT!". The apiUrl
string specifies the endpoint for the completions. The payload
string is a JSON object containing the prompt and other parameters, such as the maximum number of tokens to generate and model to use. In our case we are using "Text-Davinci-003" model and allow 100 Max Tokens.
The HTTPClient
object is then initialized, and the begin()
function is used to specify the API endpoint URL.
Next, we add the HTTP headers, such as Content-Type where we specify that we will communicate using JSON data and Authentication header to authenticate to ChatGPT API with the API_KEY.
- Finally we use the
http.POST()
function to send the HTTP POST request to the OpenAI API service.
int httpResponseCode = http.POST(payload);
if (httpResponseCode == 200) {
String response = http.getString();
// Parse JSON response
DynamicJsonDocument jsonDoc(1024);
deserializeJson(jsonDoc, response);
String outputText = jsonDoc["choices"][0]["text"];
Serial.println(outputText);
} else {
Serial.printf("Error %i \n", httpResponseCode);
}
The http.POST()
will return the response HTTP code. In case it is HTTP 200, we will parse the JSON and print it to Serial Port.
In case, other HTTP code than 200 is returned, we will print "Error: HTTP CODE", for example if your API token is not valid, it will print "Error: 401".
- We need to provide the
loop()
function. It does nothing, but it is simply a placeholder for any other code that might be added to the program in the future.
void loop() {
//
}
And the final result should look like this: #
#include <WiFi.h>
#include <HTTPClient.h>
#include <ArduinoJson.h>
// Replace with your network credentials
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
// Replace with your OpenAI API key
const char* apiKey = "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";
void setup() {
// Initialize Serial
Serial.begin(9600);
// Connect to Wi-Fi network
WiFi.mode(WIFI_STA);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
Serial.print("Connecting to WiFi ..");
while (WiFi.status() != WL_CONNECTED) {
Serial.print('.');
delay(1000);
}
Serial.println(WiFi.localIP());
// Send request to OpenAI API
String inputText = "Hello, ChatGPT!";
String apiUrl = "https://api.openai.com/v1/completions";
String payload = "{\"prompt\":\"" + inputText + "\",\"max_tokens\":100, \"model\": \"text-davinci-003\"}";
HTTPClient http;
http.begin(apiUrl);
http.addHeader("Content-Type", "application/json");
http.addHeader("Authorization", "Bearer " + String(apiKey));
int httpResponseCode = http.POST(payload);
if (httpResponseCode == 200) {
String response = http.getString();
// Parse JSON response
DynamicJsonDocument jsonDoc(1024);
deserializeJson(jsonDoc, response);
String outputText = jsonDoc["choices"][0]["text"];
Serial.println(outputText);
} else {
Serial.printf("Error %i \n", httpResponseCode);
}
}
void loop() {
// do nothing
}
Troubleshooting #
In case you encounter issues, here are the things you double check:
- Make sure you have correctly entered your network credentials, OpenAI API key, and input prompt string in the code.
- Check your Wi-Fi network connectivity. Make sure your ESP32 is connected to the correct Wi-Fi network and that it has a strong signal.
- Check that you have included all necessary libraries in your Arduino IDE. The code requires the following libraries: WiFi, HTTPClient, and ArduinoJson.
- Verify that the API endpoint URL is correct and that it is accessible from your network.
- Check that the payload for the HTTP POST request is properly formatted, including the prompt string and any other parameters.
- The
DynamicJsonDocument
object in this code is used to store the JSON response from the OpenAI API. Be aware that the size of the response must fit within the capacity of theDynamicJsonDocument
object, which is set to 1024 bytes in this example. If the response is larger than the capacity of theDynamicJsonDocument
object, it will result in a parsing error.
Since the response for our provided prompt is likely to be smaller than 1024 bytes, this size of DynamicJsonDocument
should be sufficient for this specific example. However, if the response is larger, the DynamicJsonDocument
object should be sized accordingly. The size of the DynamicJsonDocument
object should be at least equal to the size of the response to ensure that the JSON can be parsed correctly.
Alternatively, if the response is too large to fit within the capacity of the DynamicJsonDocument
object, the user can switch to a StaticJsonDocument
object, which has a fixed capacity determined at compile time.
If you are still having problems, try only connecting to the WiFi. You can find instructions for simple WiFi connection in our Hello World Code Examples post.
ChatGPT in ESP32 using the ESP-IDF #
In case you prefer to program your ESP32 with ESP-IDF and not the Arduino Core, the steps and logic are exactly the same, but there are changes due to the different framework. Please refer to ESP-IDF documentation.
If you encounter any issues, please refer to the Troubleshooting section in the previous example, using Arduino IDE.
In case you chose the later example that uses the ESP-IDF framework, and happen to have the newer version of ESP32, such as ESP32-C5, we highly recommend considering using the 5 GHz WiFi. You can find the instructions in our blog post "Can ESP32 Connect to 5GHz Wi-Fi? Exploring Wi-Fi Connectivity Options with ESP32".
Next steps and considerations #
To use this example in a real-world application, the code would need to be modified to include some sort of a user input, such as a microphone or text input. The logic for sending requests to the ChatGPT API should be moved from the setup function to the loop function to allow for continuous communication with the API.
However, there are also some challenges when using ChatGPT with an ESP32. One of the main challenges here could be the processing power needed. ChatGPT requires a significant amount of computational resources, and the ESP32's limited processing power may not be sufficient to handle more complex queries or larger text completions.
Conclusion #
Using OpenAI API to introduce similar functionalities as the ChatGPT in ESP32 projects, can provide an easier way to create more sophisticated applications, especially, if it needs some language and context understanding - such as chat bots, voice assistants, etc.
In this post, we have provided an example on how to use the OpenAI API in ESP32 microcontroller to get the benefit of the trending natural language processor. We went through step-by-step instructions on calling the OpenAI API from ESP32, using the Arduino IDE together with Arduino Core library for ESP32. Additionally, we have provided a similar example, using the ESP-IDF framework for the ones, that prefer this solution better.
Using ChatGPT with ESP32 does come with its challenges, such as the need for high processing power, but the benefits of using ChatGPT with ESP32 outweighs the challenges, especially when considering its potential for enhancing the user experience of IoT devices.
Additional Resources #
Apart from the external resources, mentioned above, here are a few more, that you might be interested in:
- 🔗 OpenAI GPT-3 Playground: This is a web based playground, where you can experiment with OpenAI's GPT-3 model, which can give you a better understanding of how to interact with the API.
- 🔗 ChatGPT chatbot: Chatbot with web interface, that was launched in November 2022, as has gained a lot of popularity since then.